🚪ox_doorlock usage
Unfortunately, ox_doorlock does not provide functions that will help us grant doorlock access to a member of the organization, so one solution may be to use it as follows.
Go to
pp-orgpanel/server/editable/framework
and find this
RegisterNetEvent('esx:playerLoaded', function(player, xPlayer, isNew)
[...]
end)
Change this part of code to this
RegisterNetEvent('QBCore:Server:PlayerLoaded', function(player)
local playerId = player.PlayerData.source
local playerIdentifier = player.PlayerData.citizenid
MySQL.Async.fetchAll('SELECT org_id FROM organization_members WHERE user_id = @identifier', {
['@identifier'] = playerIdentifier
}, function(membersResult)
if membersResult and #membersResult > 0 then
local orgId = membersResult[1].org_id
MySQL.Async.fetchAll('SELECT doorlock FROM organization_interiors WHERE org_id = @org_id', {
['@org_id'] = orgId
}, function(interiorsResult)
if interiorsResult and #interiorsResult > 0 then
local orgDoorlock = interiorsResult[1].doorlock
Player(playerId).state.orgDoorlock = orgDoorlock
end
end)
end
end)
end)
Below the
PlayerLoaded
event, add something like this to keep the door lock system in sync.
RegisterNetEvent('pp-orgpanel:memberAdded', function(orgId, sourceId, targetId)
MySQL.Async.fetchAll('SELECT doorlock FROM organization_interiors WHERE org_id = @org_id', {
['@org_id'] = orgId
}, function(interiorsResult)
if interiorsResult and #interiorsResult > 0 then
local orgDoorlock = interiorsResult[1].doorlock
Player(targetId).state.orgDoorlock = orgDoorlock
end
end)
end)
RegisterNetEvent('pp-orgpanel:memberFired', function(targetId)
Player(targetId).state.orgDoorlock = nil
end)
RegisterNetEvent('pp-orgpanel:memberLeaved', function(orgId, targetId)
Player(targetId).state.orgDoorlock = nil
end)
Now go to
ox_doorlock/server/framework/qb-core
find theIsPlayerInGroup
function and replace it with this
function IsPlayerInGroup(player, filter)
local type = type(filter)
local currentOrg = Player(player.PlayerData.source).state.orgDoorlock
if type == 'string' then
for i = 1, #groups do
local data = player.PlayerData[groups[i]]
if data.name == filter or currentOrg == filter then
return true
end
end
else
local tabletype = table.type(filter)
if tabletype == 'hash' then
for i = 1, #groups do
local data = player.PlayerData[groups[i]]
local grade = filter[data.name]
if (grade and grade <= data.grade.level) or filter[currentOrg] then
return true
end
end
elseif tabletype == 'array' then
for i = 1, #filter do
local group = filter[i]
for j = 1, #groups do
local data = player.PlayerData[groups[j]]
if data.name == group or currentOrg == group then
return true
end
end
end
end
end
return false
end
Now set the interior doorlock in
pp-orgpanel/config_c/Config.interiors
to the same name as the group in the doorlock settings, for example:
['interior1'] = {
size = vec3(1.20000004768371, 0.60000002384185, 3.0),
coords = vec3(746.5399780273438, -1781.989990234375, 36.54000091552734),
rotation = 90,
doorlock = 'organization1' -- doorlock group name
}
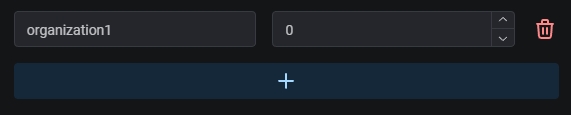
Restart your server and everything should work great now
Was this helpful?